Vue Js Disable Button for 5 Seconds:In Vue.js, to disable a button for 5 seconds, you can create a data property to keep track of the button’s disabled state, and then use a method to set that property to true
when the button is clicked. You can then use the setTimeout()
function to call another method after 5 seconds, which sets the property back to false
How can you Vue Js disable button for a specified duration, say 5 seconds, before enabling it again?
The Below code is an example of how to disable a button in Vue.js for 5 seconds. Here’s how it works:
- The HTML code defines a button element with the
disabled
attribute bound to theisDisabled
data property. This means that whenisDisabled
istrue
, the button will be disabled and cannot be clicked. - The button also has an
@click
event listener bound to thedisableButton
method. This method sets theisDisabled
property totrue
, which disables the button. - The
disableButton
method also uses thesetTimeout
function to setisDisabled
back tofalse
after 5 seconds. This means that after 5 seconds, the button will become clickable again. - Finally, the button text is conditionally rendered based on the value of
isDisabled
. IfisDisabled
istrue
, the button will display “Wait for 5 seconds…”. Otherwise, it will display “Click me”.
Overall, this code provides a simple way to disable a button for a set period of time in Vue.js.
Vue Js Disable Button for 5 Seconds Example
<div id="app">
<button :disabled="isDisabled" @click="disableButton">{{ isDisabled ? 'Wait for 5 seconds...' : 'Click me' }}</button>
</div>
<script type="module">
const app = new Vue({
el: "#app",
data() {
return {
isDisabled: false
}
},
methods: {
disableButton() {
this.isDisabled = true
setTimeout(() => {
this.isDisabled = false
}, 5000)
}
}
});
</script>
Output of Vue Js Disable Button for 5 Seconds
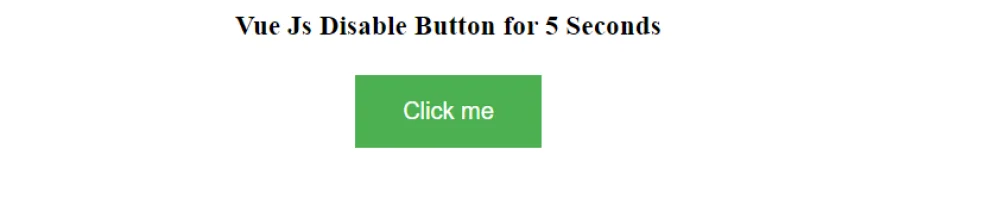